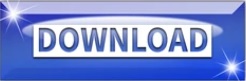
And printf has a ton of features that write() can't do. You can do an ungetc() but there is no unread(). So for each application, you need to consider which technique is faster.Īnother consideration is that stdio has a richer feature set than is available directly from the system call interface. Then it's copied from stdio's buffer into your program. Then it's copied from the buffer cache into stdio's buffer. The data is read from the disk into the buffer cache. On the other hand, if you are reading a disk file block by block, the stdio buffering will probably slow you down. Each read and write goes all the way to the driver. In C, we use a structure pointer of a file type to declare a file: FILE fp C provides a number of build-in function to perform basic file operations: fopen () - create a new file or open a existing file. If you are doing i/o to, say, /dev/tty, there is no buffer cache. File handling is one of the most important parts of programming. And that's with a disk file which is using a buffer cache. Now if you read character by character, it will go much faster. relative dirs and / with replacement work). By using stdio, one read will happen to load some data into the library's buffer. Having both the above configured, you can simply use FILE f fopen('./my-very-long-path/my-file.txt') without any limitations (e.g.
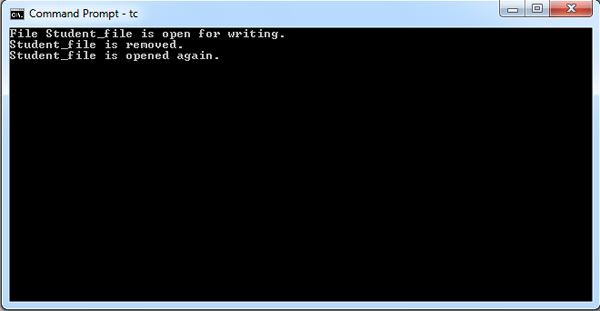
Even with a disk file, where the data is being fetched from the buffer cache, a separate read for each character will take too long. If you want to read a file character by character, issuing separate read system calls is slow. If your program is intended for unix systems only, the most important factor is i/o buffering.
#C using fopen to open any file name portable
So to write C code truely portable to non-unix systems, you must use stdio. This is not necessarily true of the unix system calls. When C is implemented on other platforms, the stdio library will be there (the ansi C standard mandates this). stdio was developed as part of the C language. stdio includes other routines like getchar, putchar, etc. Stuff like fopen, fclose, fread, etc are put of the standard I/O library or stdio. The system calls like read, write, and open must exist if programs are going to be able to use the kernel's drivers. But lots of code had been written using creat, so it persists. The mode variable contains one positional parameter followed by optional keyword parameters.
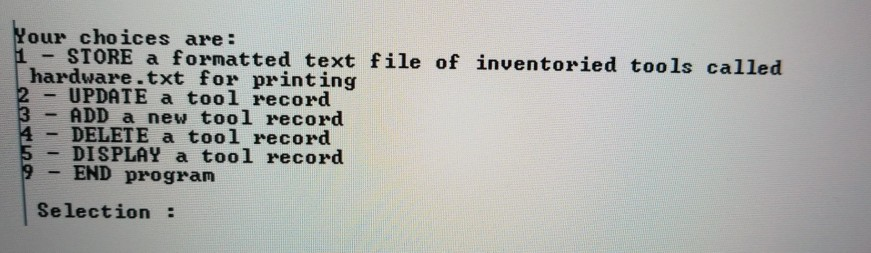
The mode parameter is a character string specifying the type of access that is requested for the file.

fopen () prototype FILE fopen (const char filename, const char mode) The fopen () function takes a two arguments and returns a file stream associated with that file specified by the argument filename. Now that you can do "open(file, O_CREAT|O_TRUNC|O_WRONLY, mode)", creat no longer has any great use. The fopen () function opens the file that is specified by filename. The fopen () function in C++ opens a specified file in a certain mode. Originally, the open system call could only open files that existed.
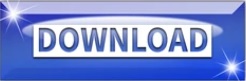